Why is JavaScript so hard?
- Risclover
- Oct 19, 2021
- 4 min read
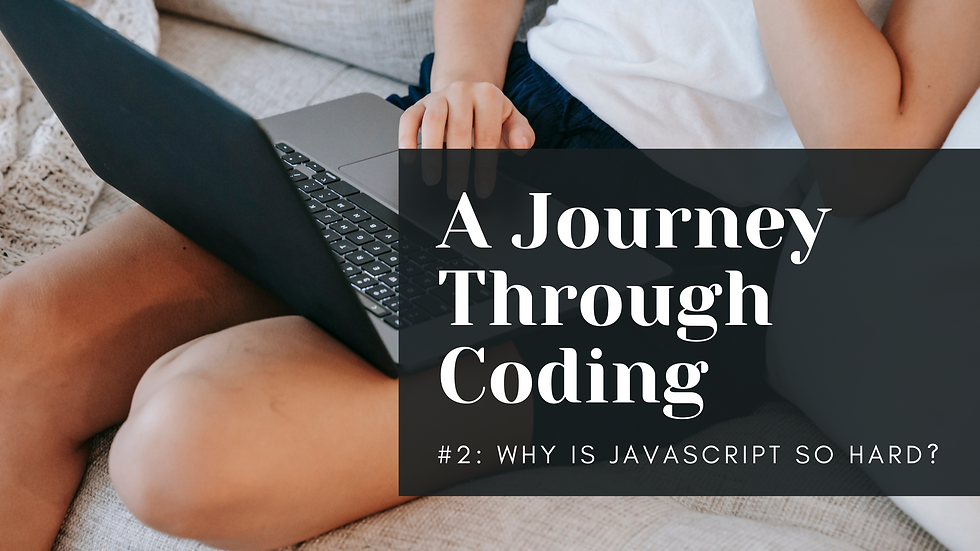
Although it took a little longer than I initially thought, I ended up learning HTML and CSS with relative ease, so I was bright-eyed and bushy-tailed about coding (and my future in it) when I decided to dip my toes into JavaScript.
And then I actually started JavaScript.
I don't know, guys. There's just something about JavaScript that totally escapes my brain. First of all, I don't even know what JavaScript does. I know it makes websites more interactive and such - that's not what I necessarily mean. I've been learning JavaScript foundations (variables, functions, arrays, etc.), but I have yet to figure out how to put any of it together to actually do something. None of the popular free coding tutorial websites are clearing that up for me as of yet. I'm frustrated.
I feel like, with coding anyway, I'm the type of learner who learns really well when actually executing the code. I've been wanting to actually build something or do something with JavaScript, but these websites (which were perfect when it came to learning HTML and CSS, by the way) aren't doing that for me.
Watch and Code
The good news is that I may have found a website that does do that for me. Just yesterday.
I discovered this website named Watch and Code, and so far, it's looking pretty promising. Although it only offers a beginner's course for free, Gordon (the teacher) is teaching in a way that I seem to understand. And I'm convinced that it's because we're building something - a Todo list. And not only are we building a to-do list, but we're also improving it along the way using different JavaScript concepts.
Recap of Watch and Code So Far
This is what I've done in this Watch and Code website so far, which also details, in part, what I've learned. (I'm finally learning stuff in JavaScript!)
Version 1
For example, we built "Version 1" of this todo list thingy first. V1 had the following requirements:
It should have a place to store todos.
It should have a way to display todos.
It should have a way to add a todo.
It should have a way to edit a todo.
It should have a way to remove a todo.
When all was said and done, this is what V1 looked like:
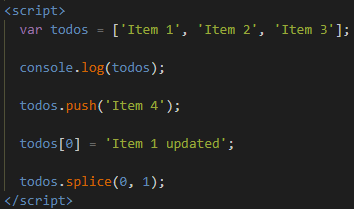
As you can see, V1 is made up of very basic JavaScript concepts/code.
Piece by piece, this is what we did:
It should have a place to store todos:
var todos = ['Item 1', 'Item 2', 'Item 3'];
Variables (var) store values. We created the variable todos and initialized it to an array, our "todo list".
It should have a way to display todos:
console.log(todos);
console.log is a function that prints something to the console. Hence, it displays our todo list.
It should have a way to add a todo:
todos.push('Item 4');
The .push method takes your string and adds it to the end of the array. So we have added 'Item 4' to our todo list.
It should have a way to edit a todo:
todos[0] = 'Item 1 updated';
This line of code focuses on the 1st item of the array (todos[0]) and updates it to a new value.
It should have a way to remove a todo:
todos.splice(0, 1)
The .splice method allows us to remove items from an Array. The first number specifies at which item we want to start, and the second number specifies how many items we want to splice total. We started at the first item, and we removed just one item.
Version 2
Following this was Version 2 (V2), which had the following requirements:
It should have a function to add a todo.
It should have a function to edit a todo.
It should have a function to remove a todo.
This is the finished V2:
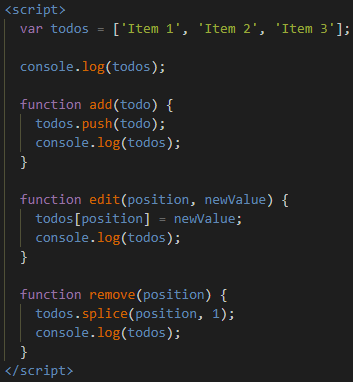
We deleted the "add", "edit", and "remove" lines of code (.push, the array update, and .splice) and replaced them with functions to meet our new requirements.
It should have a function to add a todo.
function add(todo) {
todos.push(todo);
console.log(todos);
}
We created a function called add with one parameter called todo.
Inside of our function, we included a .push method again (todos.push(todo);), which adds whatever string we include inside add( ); to our todo list.
We also included a console.log to print the result to the console.
It should have a function to edit a todo.
function edit(position, newValue) {
todos[position] = newValue;
console.log(todos);
}
We created a function called edit with two parameters - position and newValue.
Our next line of code is similar to the todo[0] = 'Item 1'; line of code from V1, but is updated so that we can edit any todo rather than just the item entered into the string. The position parameter in todos[position] refers to the position of the todo item (for example, todos[0]), and the newValue parameter refers to the new value you wish your todo to be edited to.
Once again, we have a console.log to print the result.
It should have a function to remove a todo.
function remove(position) {
todos.splice(position, 1);
console.log(todos)
}
Our todos.splice(0, 1); has been replaced with something a tad more complex; instead of (0, 1), we simply have (position, 1).
position is the position of the todo list item that we wish to remove. The 1 remains because we only wish to remove 1 item at a time.
And as always, we have console.log so that the result is printed to the console.
Takeaway
I love this website. I posted all of the above because it helps me retain what I've been learning (and typing it out helps me figure out what I'm learning in the first place). At first while building "Version 2" I was pretty confused, but upon revisiting it all again for this blog post, I totally get all of the code that we wrote for this, and that makes me excited!
I plan to continue to write about my "adventures in coding" - what I'm doing, what I'm learning, etc. If anything, writing about it and summarizing it helps ingrain everything into my brain, which is definitely needed (especially for this damned JavaScript!).
Comentários