My first JS "program" from scratch!
- Risclover
- Oct 27, 2021
- 6 min read
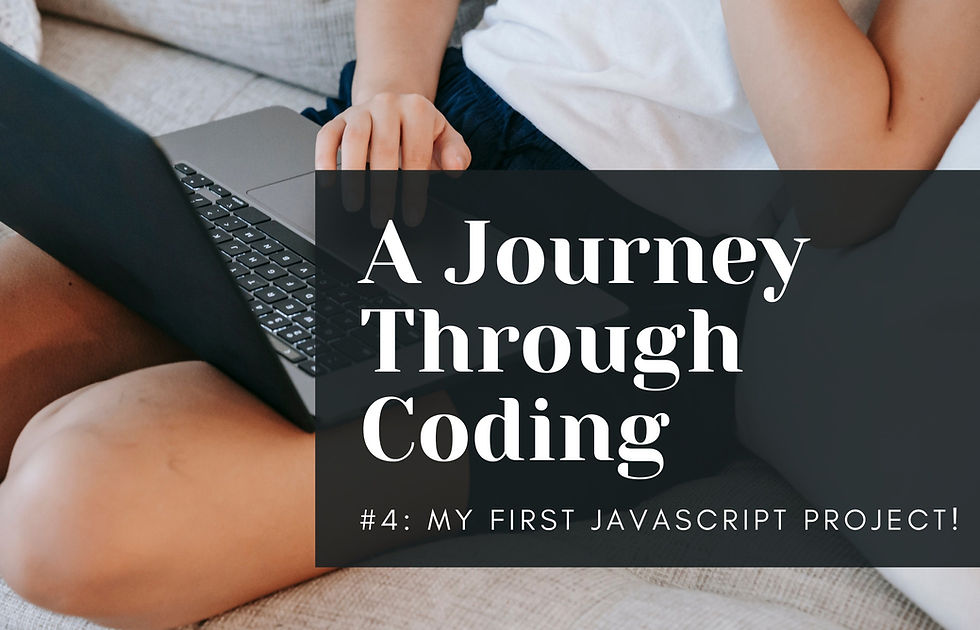
Ahhh! I wrote my first JavaScript program! Well, I mean, not my FIRST JS program… but it's my first JS program that I wrote from scratch, after coming up with my own idea!
…sort of. I did need a little help with it. Allow me to explain.
Earlier
I was working on a Mozilla Developer JavaScript assessment, which asks you to do the following:
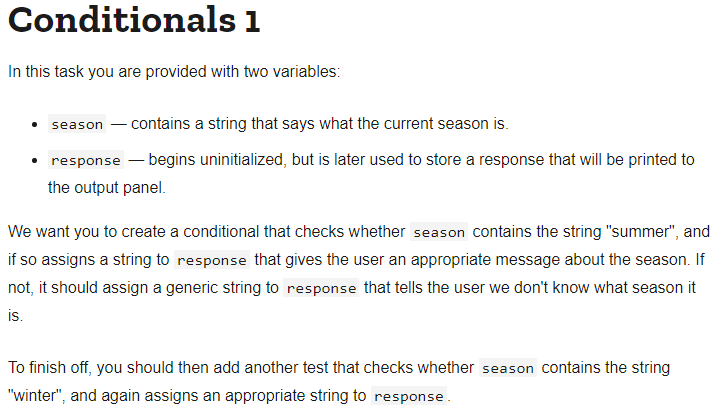
I was able to do this fairly easily:
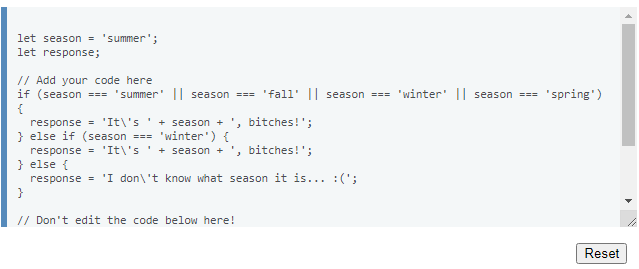
But this project gave me the random idea of creating a JS program that consists of nothing more than an input text box and a submit button. If you enter text that the program identifies as a season (Winter, Spring, Summer, or Autumn/Fall), it returns with a "validation message" that says "It's (season), bitches!". If you enter text that isn't a season, it returns with a different message that says "I don't know what season it is." Sounds pretty simple, right? I remembered seeing some examples from W3Schools where, upon clicking a button, text would pop up on the screen.
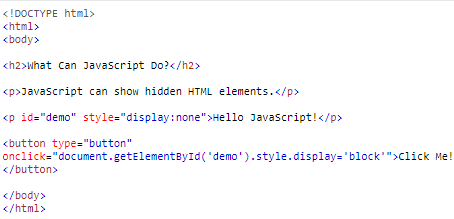
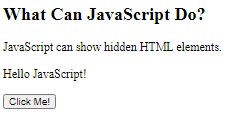
That isn't exactly what I needed here, but I figured a combination of the Mozilla Developer code and the W3 Schools code could bring me close to what I needed. And I was right, in a way. After about an hour of trial and error (and some Googling for help), this is what I finally figured out.
The Result
This is what the final product looks like:

Don't get me wrong, it isn't anything beautiful or special. It's just simply what the thing looks like. And of course, you can't tell what this program does from this screenshot alone, but don't worry - we'll get to that soon!
As with many (if not all) web- or browser-based projects, the HTML is necessary in order for you to be able to test and showcase your JS program, so let's first start with the HTML code.
HTML
<body>
<header>
<h1>Season Checker</h1>
<p id="subtitle">
See what season it is using our patented Season Checker!
</p>
</header>
<main>
<div id="main">
<form id="seasonChecker" action="">
<label for="checkerbox">Enter season:</label>
<input type="text" id="checkerbox" placeholder="Ex: Winter">
<button type="button" onclick="myFunction()">Submit</button>
</form>
</div>
<p id="results"></p>
</main>
<script src="seasons.js"></script>
</body>
For those of you who aren't well-versed with HTML code, let's quickly go through the code bit by bit.
My HTML code consists of two main parts - the header (wrapped in <header> tags) and the main part (wrapped in <main> tags).
Header
<header>
<h1>Season Checker</h1>
<p id="subtitle">
See what season it is using our patented Season Checker!
</p>
</header>
This is my header, which consists of just two elements.
<h1>Season Checker</h1>
The <h1> tag creates my heading (content title)...
<p id="subtitle">
See what season it is using our patented Season Checker!
</p>
and the <p> tag (with the ID of "subtitle") is, well, my subtitle, of course!
Main
<main>
<div id="main">
<form id="seasonChecker" action="">
<label for="checkerbox">Enter season:</label>
<input type="text" id="checkerbox" placeholder="Ex: Winter">
<button type="button" onclick="myFunction()">Submit</button>
</form>
</div>
<p id="results"></p>
</main>
Following the header, we have the "main" part of my page, which also contains just a few parts.
Note that, apart from the last element (the final <p> element), everything else is wrapped in a <div> element, which I want to clarify was just for possible styling purposes. It wasn't actually needed in the end.
<form id="seasonChecker" action="">
<label for="checkerbox">Enter season:</label>
<input type="text" id="checkerbox" placeholder="Ex: Winter">
<button type="button" onclick="myFunction()">Submit</button>
</form>
We have a form, which is just the <input> text box, a <label> for that text box, and a submit <button>. The label says "Enter Season:", the input text box has a placeholder of "Ex: Winter", and the submit button says "Submit".
<p id="results"></p>
And last of all is our <p> tag, which is empty (without text) because we don't want text, apart from what the JavaScript code will generate.
Take notice of the various ID attributes in these HTML elements. The ones worth mentioning are the IDs for <input> ("checkerbox") and <p> ("results").
Also worth mentioning is the onclick=" " attribute that the <button> element has, which has the value of myFunction( ):
<button type="button" onclick="myFunction()">Submit</button>
These IDs will come into play a little bit later, so just keep them in the back of your mind.
CSS
The CSS code styles the appearance of the page. I didn't need much styling, and I didn't want to necessarily waste my time with a whole bunch of styling when my real goal was to make a quick program, just to see if I could do it. But since it's part of it, here's the CSS code:
body, html {
font-family:'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
h1 {
margin-bottom: 0;
padding: 0;
text-align: center;
font-size: 2em;
}
#subtitle {
font-size: 1em;
text-align: center;
font-style: italic;
margin: 0;
padding: 0 0 50px 0;
}
main {
display: flex;
margin-left: auto;
margin-right: auto;
width: 100%;
}
#seasonChecker {
display: flex;
flex-direction: column;
justify-content: space-between;
width: 100%;
padding: 20px;
margin-left: 280px;
}
form {
width: 100%;
}
label {
margin-bottom: 2px;
font-weight: bold;
}
input {
width: 200px;
height: 25px;
margin: 5px 0;
border: 1px solid gray;
border-radius: 3px;
padding: 0 5px;
}
button {
margin: 5px 0;
height: 25px;
border-radius: 3px;
border: 1px solid gray;
width: 75px;
cursor: pointer;
}
button:hover {
background-color: pink;
}
button:active {
background-color: rgb(213, 131, 144);
}
JavaScript
And finally, the JavaScript code!
function myFunction() {
let input = document.getElementById("checkerbox").value;
let text;
if (input === 'Spring' || input === 'Winter' || input === 'Fall' || input === 'Autumn' || input === 'Summer') {
text = 'It\'s ' + input + ', bitches!';
} else {
text = 'I have no idea what season it is. Damn.';
}
document.getElementById("results").innerHTML = text;
}
Quite a bit smaller than the HTML and CSS codes, isn't it?
Just as we did with the HTML code, let's go through the JavaScript code bit by bit. It begins like so:
function myFunction() {
...
}
The whole thing is nothing more than a function, which I "creatively" decided to name myFunction.
let input = document.getElementById("checkerbox").value;
let text;
The first thing I had to do within myFunction was to create my variables.
The first variable, input, is initialized to document.getElementById("checkerbox").value;. The document object is followed by the .getElementById(" ") method, which (in general) returns the element that has the ID attribute with the specified value. If you'll recall, the element with the checkerbox ID was my input text box. So
The final bit is .value, which sets or returns the value of the value attribute of a text field.
Altogether, this line of code sets the variable input to a command that says "get the value of the input text box with the ID of checkerbox". In other words, when text is entered into the text box, this line of code retrieves it.
We also needed another variable (let text;). We haven't made it equal to anything because we need to do that a little later on; for now, we just needed to establish its existence.
After setting my variables, I created an if/else condition. An if/else condition is something that, in very broad terms, says "If x is true, execute y, and if not, execute z".
My if/else statement begins like this:
if (input === 'Spring' || input === 'Winter' || input === 'Fall' || input === 'Autumn' || input === 'Summer') {
There's that input variable from earlier, which, if you'll recall, represents the text entered into the text box.
| | means "or", and === means "equals to". So what this line of code is saying is "If the text inputted into the box equals Spring, Winter, Fall, Autumn, or Summer, then execute the true code."
This is our so-called "true" code:
text = 'It\'s ' + input + ', bitches!';
Remember our text variable from earlier? We're initializing it now. So if the text entered equals any of the aforementioned season values, then text will equal the string 'It's (input), bitches!'.
Since input would be the name of a season in this case, the text would be something like "It's Winter, bitches!", or "It's Summer, bitches!" (and so on).
So that's our "true" code. What about our "false" code?
} else {
text = 'I have no idea what season it is. Damn.';
}
As you can see, we have } else {, which is the "if not" part of our broad explanation of if/else statements from earlier. So this code is saying "And if the inputted text doesn't equal any of the seasons, then execute the false code."
Our false code is, of course, text = "I have no idea what season it is. Damn.". So instead of displaying "It's input, bitches!", the text shown will be "I have no idea what season it is. Damn."
And that wraps up our if/else condition!
The last part of our function is the following:
document.getElementById("results").innerHTML = text;
There's that document.getElementById(" ") again, but this time, the ID is "results", which was the ID of the last <p> element in my HTML code.
This is followed by .innerHTML, which is a method that sets or returns the HTML content (inner HTML) of an element. This method is set equal to the content you want to show, which in this case is whatever is in the text variable!
Remember this line of code back in our HTML?:
<button type="button" onclick="myFunction()">Submit</button>
As you can see, the <button> element's onclick attribute is set to myFunction, the name of my function. So what this bit is saying is "When this button is clicked, run myFunction". And when myFunction runs, it tests whether or not the text entered into the input box is a season or not. If it is, it displays a line of text, and if it isn't, then it displays a different line of text.
Here's my little "Season Checker" program working:

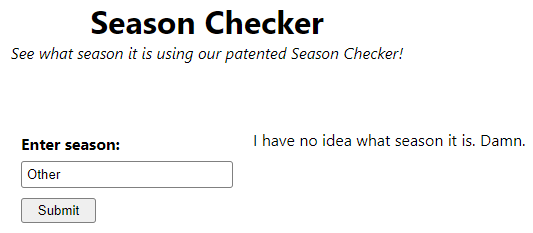
Comments